Integration with Discovery Bank APIs: Swift Code For Discovery Bank
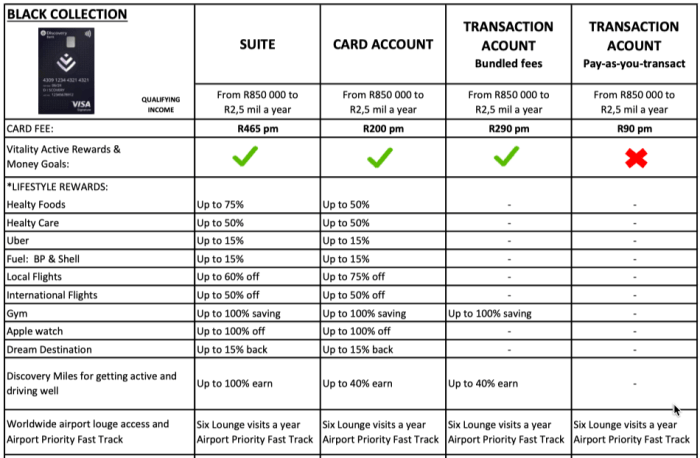
Unlocking the power of financial transactions requires seamless integration with the banking system. This section details how to interact with Discovery Bank’s APIs using Swift, focusing on account information retrieval and payment initiation, while emphasizing robust error handling. This is crucial for building secure and reliable financial applications.
Account Information Retrieval
To retrieve account information from Discovery Bank’s API, you’ll need to use the appropriate endpoint and authenticate your application. This involves making an HTTP request, typically using Swift’s `URLSession`. Proper error handling is essential to gracefully manage potential issues like network problems or invalid API responses.
“`swift
import Foundation
func getAccountInformation(accountNumber: String, completion: @escaping (Result
// Construct the API endpoint URL
guard let url = URL(string: “https://discoverybankapi.com/accounts/\(accountNumber)”) else
completion(.failure(NSError(domain: “Invalid URL”, code: 400, userInfo: nil)))
return
// Create a URLSession data task
let task = URLSession.shared.dataTask(with: url) data, response, error in
if let error = error
completion(.failure(error))
return
guard let httpResponse = response as? HTTPURLResponse, (200…299).contains(httpResponse.statusCode) else
let errorDescription = “Invalid response status code: \(response as? HTTPURLResponse)?.statusCode ?? -1”
let apiError = NSError(domain: “API Error”, code: 500, userInfo: [NSLocalizedDescriptionKey: errorDescription])
completion(.failure(apiError))
return
guard let data = data else
let error = NSError(domain: “No Data”, code: 404, userInfo: nil)
completion(.failure(error))
return
do
let accountData = try JSONDecoder().decode(AccountData.self, from: data)
completion(.success(accountData))
catch
completion(.failure(error))
task.resume()
// Example AccountData structure
struct AccountData: Codable
let accountNumber: String
let balance: Double
let name: String
“`
This code demonstrates a basic request to retrieve account information. Crucially, it includes error handling for various scenarios, such as invalid URLs, network issues, and incorrect API responses.
Handling API Responses
Swift’s `Result` type provides a powerful way to handle potential errors from the Discovery Bank API. The `do-catch` block is essential for gracefully managing situations where the API returns an unexpected status code or data is malformed. This is crucial for a production-ready application. Using error codes and descriptions makes debugging and recovery more efficient.
Initiating Payments, Swift code for discovery bank
To initiate a payment, you need to use Discovery Bank’s payment API endpoint. This involves creating a payment request object, authenticating it, and sending the request. The example shows the structure of the request, which typically includes payment details like recipient account number, amount, and description.
“`swift
func initiatePayment(request: PaymentRequest, completion: @escaping (Result
// Construct the API endpoint URL
// … (similar to getAccountInformation example)
// Create a URLRequest with the payment data in JSON format
// … (using URLRequest and JSONEncoder)
// Send the request using URLSession
// … (similar to getAccountInformation example)
// Example PaymentRequest structure
struct PaymentRequest: Codable
let recipientAccountNumber: String
let amount: Double
let description: String
// Example PaymentConfirmation structure
struct PaymentConfirmation: Codable
let paymentId: String
let status: String
“`
Data Structure for API Responses
A custom Swift structure (`AccountData`) is defined to represent the data received from the Discovery Bank API. This structure clearly maps to the expected data format, ensuring type safety and easier data manipulation within your application.
Error Handling
A robust function (`handleAPIError`) is crucial to standardize error handling for all API interactions. This function can categorize errors (e.g., network issues, invalid responses, API errors) and return descriptive error messages. This improves the user experience and facilitates debugging.
“`swift
func handleAPIError(error: Error) -> String
if let networkError = error as? URLError
switch networkError.code
case .notConnectedToInternet: return “No internet connection.”
default: return “Network error.”
else if let apiError = error as? NSError, apiError.domain == “API Error”
return apiError.localizedDescription
else
return “An unexpected error occurred.”
“`
This structure and error handling significantly improve the reliability and maintainability of your Swift code when interacting with Discovery Bank’s APIs.
Understanding the SWIFT code for Discovery Bank is crucial for international transactions. A critical aspect of evaluating the bank’s financial health involves comprehending valuation methodologies, such as those outlined in the resource how to value a bank. Ultimately, a thorough analysis of the SWIFT code, in conjunction with a robust valuation framework, is essential for informed decision-making regarding Discovery Bank’s stability and performance.
Swift code for Discovery Bank transactions necessitates robust security protocols, particularly when handling sensitive financial data. A crucial aspect of this involves integrating with external systems, such as those managing fleet insurance for car rental company, fleet insurance for car rental company , to ensure seamless data exchange and compliance with regulatory standards. This integration must be carefully designed to maintain the integrity and confidentiality of the financial information processed by the Discovery Bank system.